Introduction
Debugging StackOverflow Exception is one of the most common problems that all developers come across during their careers, it is classified as “Fatal Error” and a non-recoverable scenario and the CLR (Common Language Runtime) forces the application to shut down which means we cannot enclose this line of code in a Try/Catch block either.
So How do we debug the Stack Overflow error? This will be our topic for today
📌 Outline
- What is Stack Overflow?
- Debugging Stack Overflow Exception
📌 Prerequisites
- Visual Studio 2022 IDE (Community/Professional/Enterprise)
What is Stack Overflow?
Before we go for a deeper dive, First we must know what Stack Overflow is and as we know it is made of two separate words “Stack” and “Overflow”
In this case, Stack refers to a structure that holds information regarding function calls and their parameters, and Overflow refers to the case when the stack cannot hold more data. So simply put, when a stack cannot accommodate more function calls and parameters, an exception is thrown and that exception is called Stack Overflow. To learn more, take a look at Microsoft’s Documentation
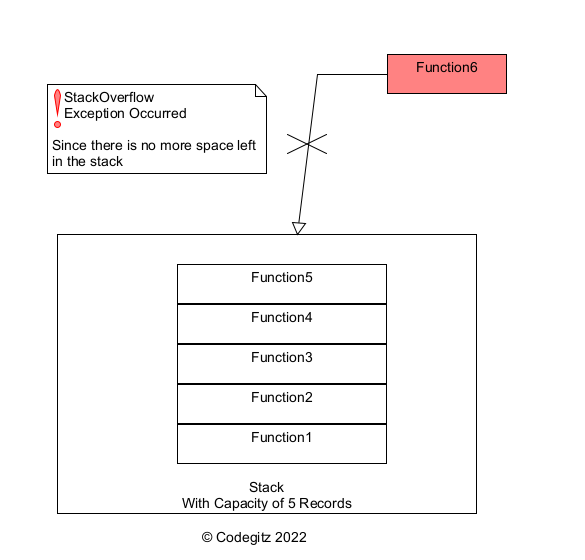
The diagram above consists of a Stack which can hold the information of up to 5 functions and their parameters, however once the 6th record is pushed on to the stack that’s when the Stack Overflow exception is thrown as there is no more room for accommodation
Debugging Stack Overflow Exception?
Now to locate the problem and find the solution
âš¡ Problem
To understand the cause of this Exception, we will take a look at a few code examples first
Recursive Case
namespace CodeGitz { internal class Program { static void FunctionCall() { FunctionCall(); } static void Main(string[] args) { FunctionCall(); } } }
Circular Dependency
namespace CodeGitz { internal class Program { static void FunctionA() { // Do Work // Dependency on Function B FunctionB(); } static void FunctionB() { // Do Work // Circular Dependency on Function A FunctionA(); } static void Main(string[] args) { FunctionA(); } } }
So what’s the denominator here? What is common between the two examples? UNBOUNDED AND DEEP RECURSION because these two examples have no base case and keep calling those functions INDEFINITELY until the STACK RUNS OUT OF MEMORY TO ALLOCATE
🛠Solution
So now that we discovered what causes the Exception, How do we locate the culprit? We will use one of the debugging tools provided by Visual Studio IDE known as “Call Stack”.
The “Call Stack” can be found in the bottom-right corner of the IDE, as marked in the screenshot below.
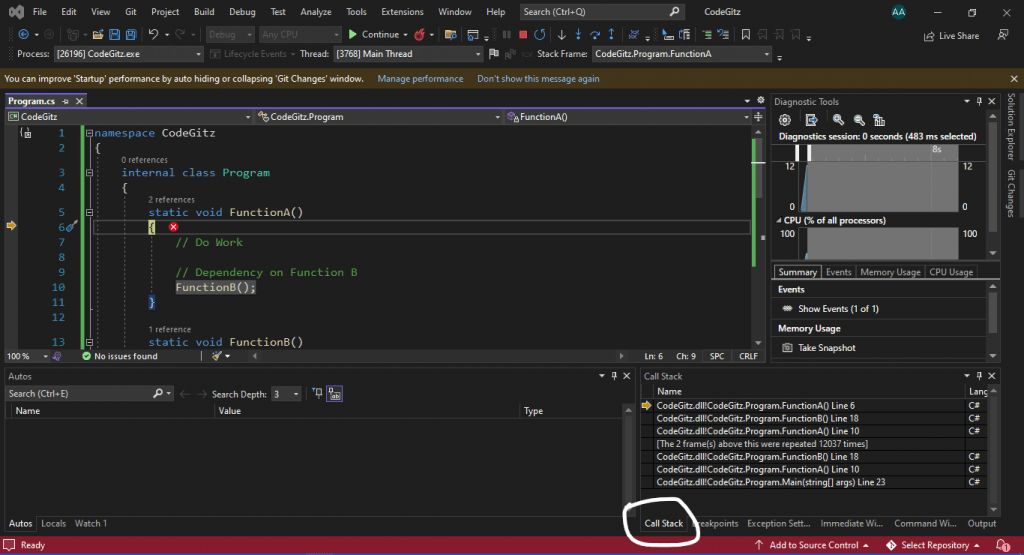
Now that we know what to use for debugging, How exactly does one utilize the “Call Stack”? The answer is simple.
- Navigate to the “Call Stack” tab
- Start from top to bottom and look for the row that states
[The N frame(s) above this were repeated X times]
- Frames mean the function calls / blocks of code that executed X times, which means this is the defected code
- Click on those frames above, and you’ll quickly realize where the problem is
Example
For this example, we will refer to the “Circular Dependency” example in section 3.1.2
- Execute the code in section 3.1.2
- Stack Overflow exception is thrown, and you’ll come across this popup

- Navigate to the bottom right of your Visual Studio IDE and click “Call Stack” tab
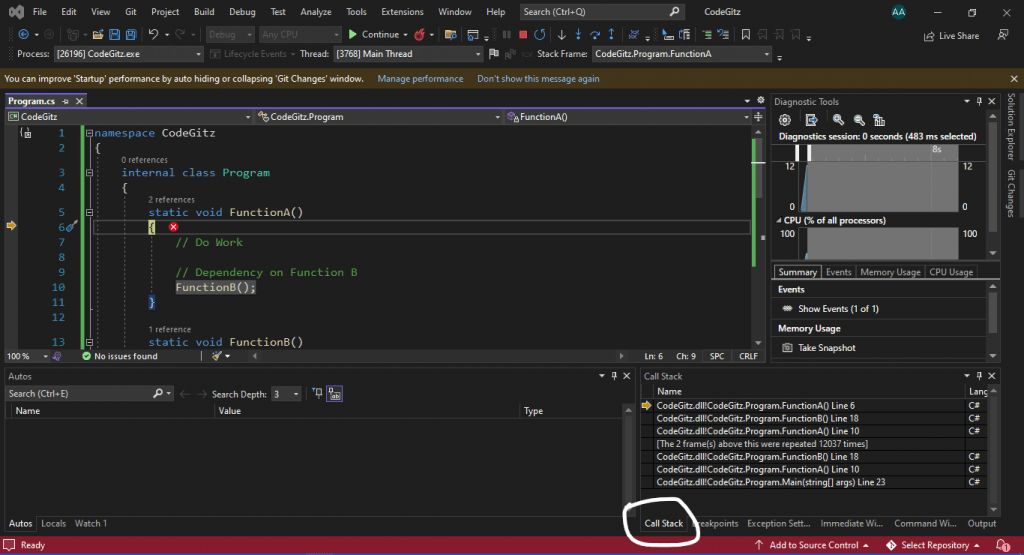
- Starting from top to bottom, look for the row that starts with “[The N frame(s) …”
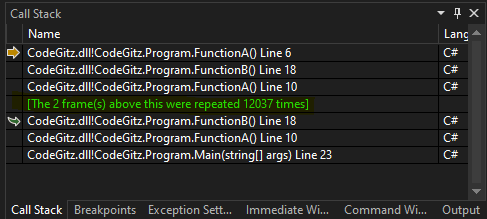
- Above this line are the defected blocks of code and following the Stack trace we can conclude that in case of this example, It is Function A and Function B with Circular Dependency that causes the Stack Overflow exception
static void FunctionA() { // Do Work // Dependency on Function B FunctionB(); } static void FunctionB() { // Do Work // Circular Dependency on Function A FunctionA(); }
Conclusion
Before we conclude, let’s take a quick recap. We learned that,
- Stack Overflow exception is caused when the Stack runs out of memory and no more function calls can be accommodated for the program Stack.
- Stack Overflow is caused by functions with Infinite Recursive Calls or Circular Dependency between two or more functions.
- Stack Overflow’s root cause can be traced with the help of “Call Stack” debugging tool provided by Visual Studio as it contains the stack trace.
For more content like this, Visit: C# Archives