Introduction
GitHub Access Token is the only way to access a repository when sensitive files are stored in a private repository. There are many intuitive tutorials on the web for some programming languages, but not everyone addresses the C# .NET developers. Today, we will learn how to implement Github access token for HttpClient in C#.
If you are here, I assume you know what GitHub Personal Access Tokens are and how to create them. If you would like to know how to create them then Visit The GitHub Documentation then come back to this tutorial.
📌 Outline
- Configuring HttpClient with Github Access Token Authentication
- Downloading File From Private Repo
📌 Prerequisites
- Basic knowledge of GitHub and repository navigation
- Visual Studio 2019/2022 IDE
Configuring HttpClient with Github Access Token Authentication
Follow the following steps
- Go to GitHub and Create a Private Repository
- Upload your file to the repo
- Generate Your Personal Access Token
- Copy Your File’s RAW USER CONTENT LINK which looks something like this https://raw.githubusercontent.com/YOUR_USERNAME/YOUR_REPO_NAME/REPO_BRANCH/FILE_NAME.WITH_EXTENSION (Remove everything after the FILE_NAME.WITH_EXTENSION)
- Copy your Personal Access Token
- Now Copy The Following Code Snippet and appropriately replace everything you need to replace
using System.IO; using System.Net; namespace CodeGitz___Github_Access_Token { internal class Program { static HttpClient _httpClient = new(); static void Main(string[] args) { // DOWNLOAD PATH MUST HAVE EXTENSION NAME TOO FOR EXAMPLE: readme.txt DownloadFile("YOUR_RAW_GITHUB_FILE_LINK", "YOUR_DOWNLOAD_PATH_HERE"); } private static void DownloadFile(String url,String down_path) { var github_token = "YOUR_GITHUB_TOKEN_HERE"; var url_to_git = url; var download_path = down_path; // Enter Your Github Token Here var credentials = string.Format(System.Globalization.CultureInfo.InvariantCulture, "{0}:", github_token); credentials = Convert.ToBase64String(System.Text.Encoding.ASCII.GetBytes(credentials)); // Configure HttpClient Authorization Headers _httpClient.DefaultRequestHeaders.Authorization = new System.Net.Http.Headers.AuthenticationHeaderValue("Basic", credentials); // Download The File byte[] content = Task.Run(() => { var credentials = string.Format(System.Globalization.CultureInfo.InvariantCulture, "{0}:", github_token); credentials = Convert.ToBase64String(System.Text.Encoding.ASCII.GetBytes(credentials)); _httpClient.DefaultRequestHeaders.Authorization = new System.Net.Http.Headers.AuthenticationHeaderValue("Basic", credentials); return _httpClient.GetByteArrayAsync(url_to_git).Result; }).Result; File.WriteAllBytes(download_path, content); } } }
Downloading File From Private Repo
I have this file stored in my private github repo called README.md, I am going to save it as readme.txt

This is how my directory looks like before running the code

This is how it looks after I run the code
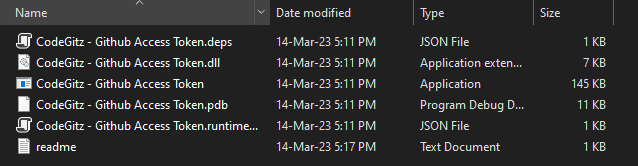
Congratulations, you have successfully downloaded a file from your private Github repository using Personal Access Token Authentication.
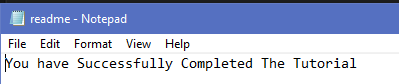
Conclusion
We learned how to authorize our HttpClient to access private GitHub repositories using GitHub’s Access Token Authentication. Then we learned how to download the file to our local drive. Now you can use this knowledge to do anything with the GitHub API or implement any other creative ideas of your own.
For more, Visit C# – Archives